Since this question was asked a year and half ago, this is for memo:
I have designed notch filter for removing 50 Hz noise but don't know how to add a 50 Hz powerline interference noise to a clean ECG signal?
In MATLAB, let's say your original signal is original_ecg
sampling_frequency = 1000;
mains_coeff = 0.1; Amplitude of mains line to change. Depends on your ECG signal.
time_step = 1/sampling_frequency;
max_time = 2; % Duration of your signal in seconds.
t = time_step:time_step:max_time; % This is our time vector.
mains_signal = cos(2*pi*60*t); % 60Hz mains frequency. Depends.
dirty_signal = original_ecg + mains_coeff*mains_signal;
plot(dirty_signal);
Here's an example signal from Physionet (100) where we add a noise of amplitude 0.1 and frequency of 60Hz. I've taken only 1second of the signal.
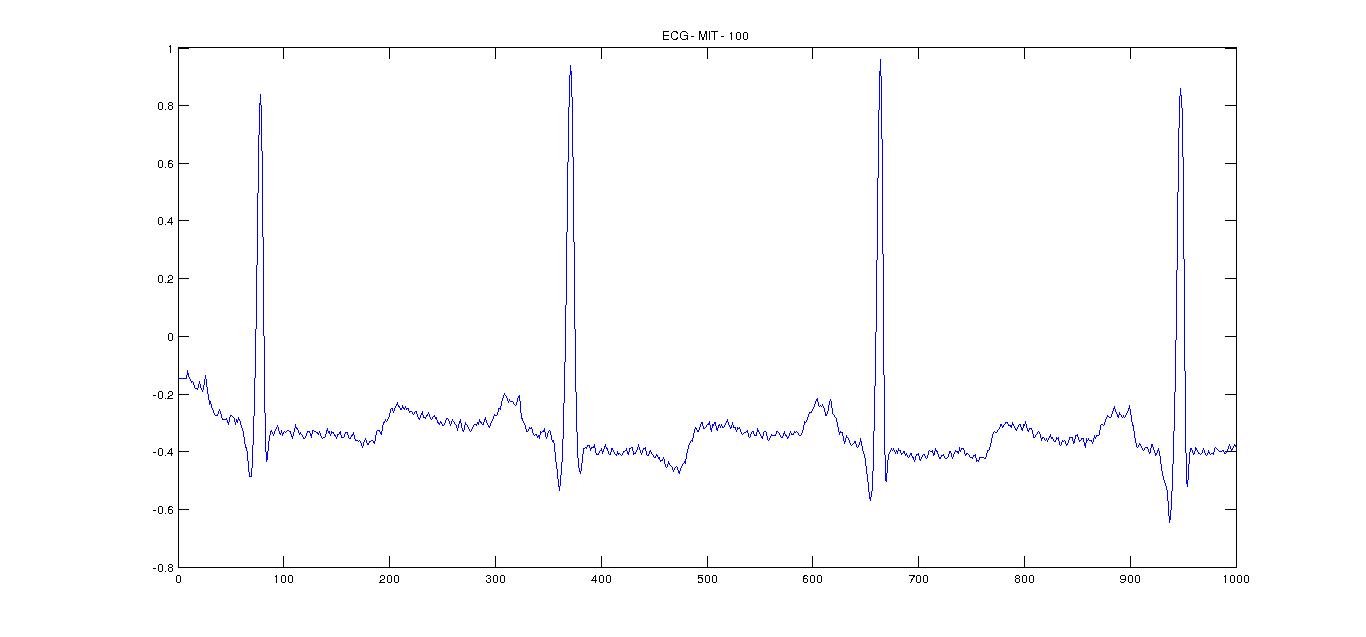
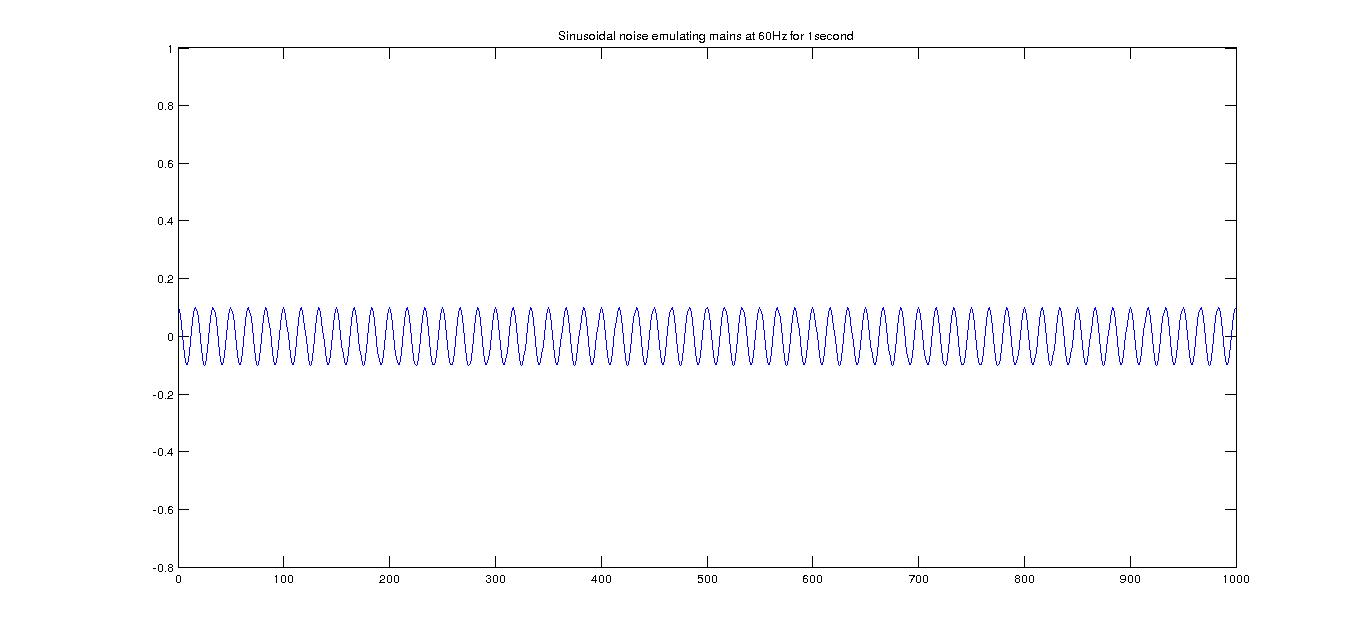
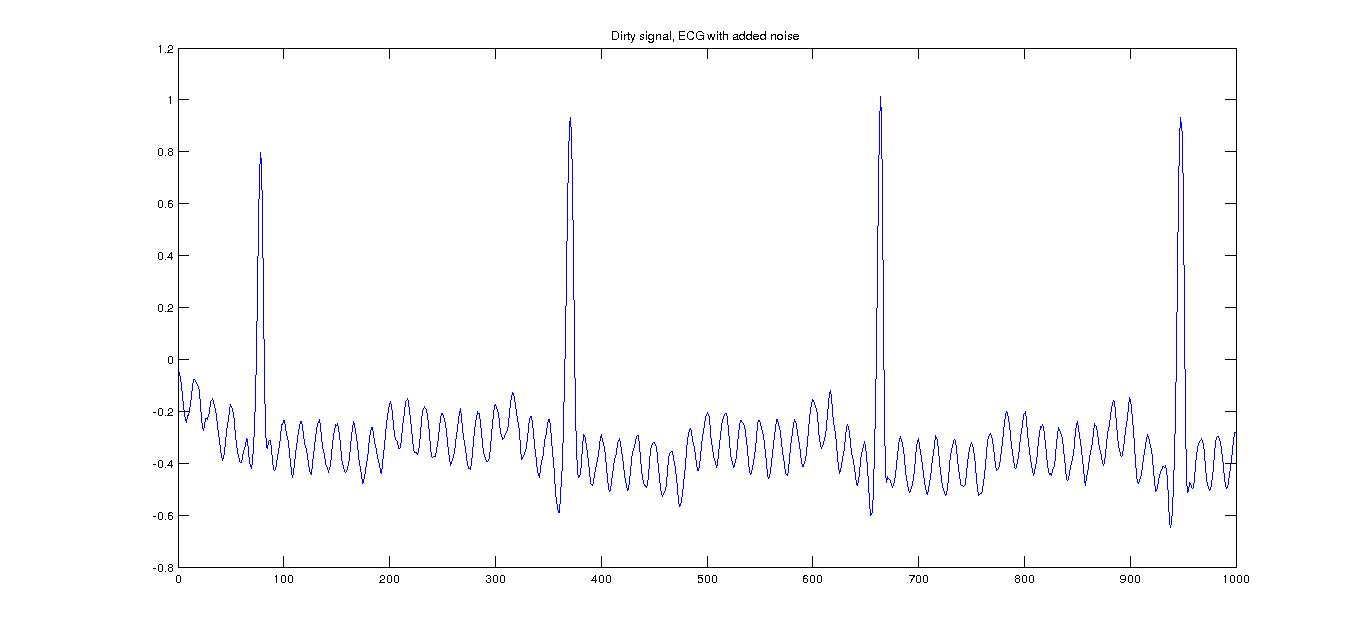
Now, I made some assumptions to explain it roughly. I arbitrarily chose a 2 seconds duration, a 1000Hz sampling frequency, a 60Hz mains power.
Important: Make sure your noise is the same length as your signal.
Meaning: If your ecg contains 100000 values, your noise should contain as much as that, or you'll get an error.
Also important: Sometimes dimensions don't match (your time is a row, so is your mains. The data you import from MIT is a column that needs to be transposed simply row_vector = column_vector'
).
Okay, you are doing PLI (Power Line Interference) filtering. In a great, perfect world, it would be a sinusoïd, but in reality, there are spikes sometimes, arcs, etc. This is a pain in the butt. Feel free to add some impulses here and there in your mains to see how your code performs.
So, to test a denoising algorithm, you add a known noise to your signal, then pass it through your algorithm to get a denoised signal, then compare between original signal and denoised signal and look at performance parameters (SNR, distortion, etc). For the PSD, you see if the frequency range you are interested in isn't attenuated, for example.
How can I compare which wavelet (e.g. db6) is best suited for ECG analysis?
Well, this is kind of tedious: You do a state of the art. You read a lot of articles about where the field is at right now. There are some good academic articles burried in a ton of crap. Unfortunately, you'll have to sort them.
A lot of articles don't contain any value actually, only an tiny incremental benefit (many times the cost is that it gets computationally intensive to the point it doesn't justify the tiny increment).
It is a business, after all, where companies want their technology and algorithm protected, so as someone said, each time someone tries to go in the field, they need to reinvent the wheel in some way or another. And where publishers want a lot of articles published (nonobstant quality, often times).
So you test, a lot. You also get inspired applying things that aren't really applied in that discipline and try things out.